Downloader使用🔥
CZerocheng 1/6/2024 Downloader文件传输
# 加载位置
Downloader 是一个现代、流畅、异步、可测试和可移植的.NET库,在Downloader的基础上加上本人常用的方式编写的使用方法。
# DownloadItem
public class DownloadItem
{
public string FolderPath { get; set; }
public string FileName { get; set; }
public string Url { get; set; }
}
1
2
3
4
5
6
2
3
4
5
6
# DownLoaderHelper
查看代码
public class DownLoaderHelper
{
public static Lazy<DownLoaderHelper> Instance => new Lazy<DownLoaderHelper>();
public static DownloadService CurrentDownloadService = null;
//下载配置参数对象,如果不配置则用默认配置参数
public static DownloadConfiguration CurrentDownloadConfiguration = null;
public void Init(DownloadConfiguration CurrentDownloadConfiguration = null)
{
if (CurrentDownloadConfiguration == null)
CurrentDownloadConfiguration = GetDownloadConfiguration();
CurrentDownloadService = new DownloadService(CurrentDownloadConfiguration);
}
public async Task DownLoadOne(DownloadItem downloadItem)
{
await DownloadFile(downloadItem).ConfigureAwait(false);
}
public async Task DownLoadMore(IEnumerable<DownloadItem> downloadList)
{
foreach (DownloadItem downloadItem in downloadList)
{
await DownloadFile(downloadItem).ConfigureAwait(false);
}
}
private async Task<DownloadService> DownloadFile(DownloadItem downloadItem)
{
if (string.IsNullOrWhiteSpace(downloadItem.FileName))
{
await CurrentDownloadService.DownloadFileTaskAsync(downloadItem.Url, new DirectoryInfo(downloadItem.FolderPath)).ConfigureAwait(false);
}
else
{
await CurrentDownloadService.DownloadFileTaskAsync(downloadItem.Url, downloadItem.FileName).ConfigureAwait(false);
}
return CurrentDownloadService;
}
private static DownloadConfiguration GetDownloadConfiguration()
{
var cookies = new CookieContainer();
cookies.Add(new Cookie("download-type", "test") { Domain = "domain.com" });
return new DownloadConfiguration
{
BufferBlockSize = 10240, // usually, hosts support max to 8000 bytes, default values is 8000
ChunkCount = 8, // file parts to download, default value is 1
MaximumBytesPerSecond = 1024 * 1024 * 10, // download speed limited to 10MB/s, default values is zero or unlimited
MaxTryAgainOnFailover = 5, // the maximum number of times to fail
MaximumMemoryBufferBytes = 1024 * 1024 * 50, // release memory buffer after each 50 MB
ParallelDownload = true, // download parts of file as parallel or not. Default value is false
ParallelCount = 4, // number of parallel downloads. The default value is the same as the chunk count
Timeout = 3000, // timeout (millisecond) per stream block reader, default value is 1000
RangeDownload = false, // set true if you want to download just a specific range of bytes of a large file
RangeLow = 0, // floor offset of download range of a large file
RangeHigh = 0, // ceiling offset of download range of a large file
ClearPackageOnCompletionWithFailure = true, // Clear package and downloaded data when download completed with failure, default value is false
MinimumSizeOfChunking = 1024, // minimum size of chunking to download a file in multiple parts, default value is 512
ReserveStorageSpaceBeforeStartingDownload = true, // Before starting the download, reserve the storage space of the file as file size, default value is false
RequestConfiguration =
{
// config and customize request headers
Accept = "*/*",
CookieContainer = cookies,
Headers = new WebHeaderCollection(), // { your custom headers }
KeepAlive = true, // default value is false
ProtocolVersion = HttpVersion.Version11, // default value is HTTP 1.1
UseDefaultCredentials = false,
// your custom user agent or your_app_name/app_version.
UserAgent = $"DownloaderSample/{Assembly.GetExecutingAssembly().GetName().Version?.ToString(3)}"
// Proxy = new WebProxy() {
// Address = new Uri("http://YourProxyServer/proxy.pac"),
// UseDefaultCredentials = false,
// Credentials = System.Net.CredentialCache.DefaultNetworkCredentials,
// BypassProxyOnLocal = true
// }
}
};
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
79
# 使用
初始化参数配置并订阅开始下载,下载进度条,下载完成时事件
public DownloaderViewModel()
{
DownLoaderHelper.Instance.Value.Init();
DownLoaderHelper.CurrentDownloadService.DownloadStarted += CurrentDownloadService_DownloadStarted;
DownLoaderHelper.CurrentDownloadService.DownloadProgressChanged += CurrentDownloadService_DownloadProgressChanged;
DownLoaderHelper.CurrentDownloadService.DownloadFileCompleted += CurrentDownloadService_DownloadFileCompleted;
}
private void CurrentDownloadService_DownloadFileCompleted(object? sender, System.ComponentModel.AsyncCompletedEventArgs e)
{
if (e.Cancelled)
{
Console.WriteLine("取消了!");
}
else if (e.Error != null)
{
Console.Error.WriteLine(e.Error.Message);
}
else
{
Console.WriteLine("下载完成!");
Console.Title = "100%";
}
}
private void CurrentDownloadService_DownloadProgressChanged(object? sender, Downloader.DownloadProgressChangedEventArgs e)
{
ProgressPercentage = e.ProgressPercentage;
}
private void CurrentDownloadService_DownloadStarted(object? sender, Downloader.DownloadStartedEventArgs e)
{
Console.WriteLine($"开始下载{e.FileName}");
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
下载单个文件
public async Task DownLoadOne()
{
await DownLoaderHelper.Instance.Value.DownLoadOne(new DownloadItem()
{
FolderPath = "D:\\TestDownload",
Url = $"http://XXXX/api/files/file-download/{Uri.EscapeDataString("D:\\Debug\\0x00\\halcon.dll")}"
});
}
1
2
3
4
5
6
7
8
2
3
4
5
6
7
8
下载多个文件
public void DownLoadMore()
{
Task.Run(async()=>
{
List<DownloadItem> downloadItems = new List<DownloadItem>();
var item1 = new DownloadItem()
{
FolderPath = "D:\\TestDownload",
Url = $"http://XXXX/api/files/file-download/{Uri.EscapeDataString("D:\\Debug\\0x00\\halcon.dll")}"
};
var item2 = new DownloadItem()
{
FolderPath = "D:\\TestDownload",
Url = $"http://XXXX/api/files/file-download/{Uri.EscapeDataString("D:\\Debug\\0x00\\halcondotnet.dll")}"
};
downloadItems.Add(item1);
downloadItems.Add(item2);
await DownLoaderHelper.Instance.Value.DownLoadMore(downloadItems);
});
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
暂停下载
public void Stop()
{
DownLoaderHelper.CurrentDownloadService.Pause();
}
1
2
3
4
2
3
4
恢复下载
public void ReSume()
{
DownLoaderHelper.CurrentDownloadService.Resume();
}
1
2
3
4
2
3
4
终止下载
public void Cancel()
{
DownLoaderHelper.CurrentDownloadService.CancelTaskAsync();
}
1
2
3
4
2
3
4
# 结果
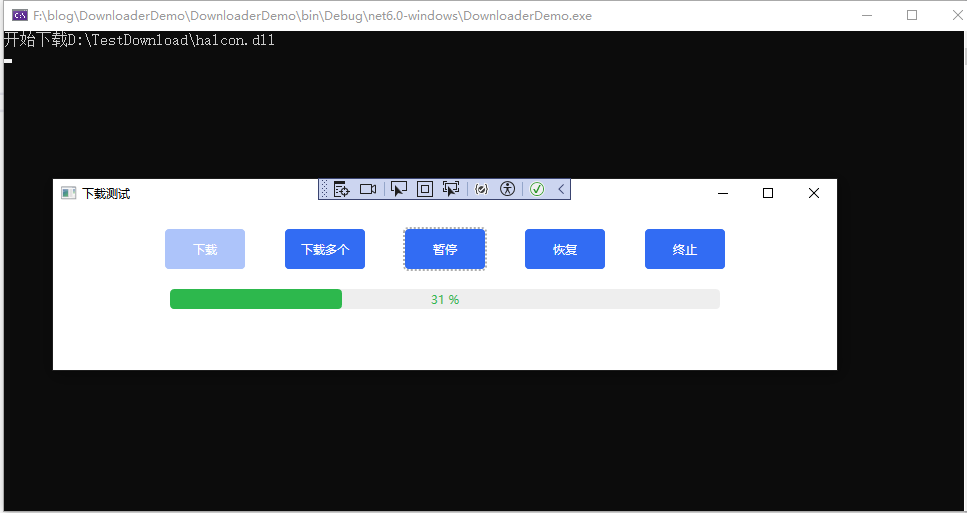