WPF之控件缩放🔥
CZerocheng 1/22/2024 WPF
# 效果展示
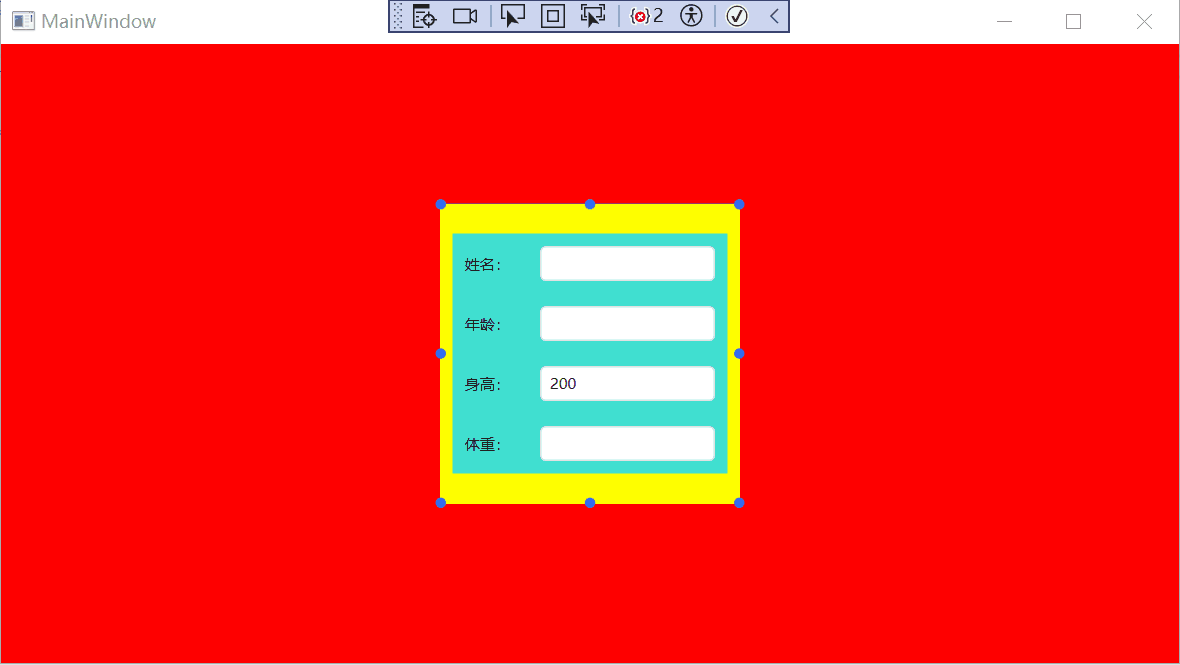
# 使用
本次示例的工程目录结构如下图所示:
其中PersonView的代码如下
<UserControl x:Class="UserControlScaleDemo.View.PersonView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:UserControlScaleDemo.View"
xmlns:hc="https://handyorg.github.io/handycontrol"
Background="Yellow"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800">
<Viewbox>
<StackPanel Margin="10" hc:BorderElement.CornerRadius="2" Background="Turquoise">
<hc:TextBox hc:TitleElement.Title="姓名:" hc:TitleElement.TitleWidth="60" Text="{Binding Name,Mode=TwoWay,UpdateSourceTrigger=PropertyChanged}" Width="200" hc:TitleElement.TitlePlacement="Left" Margin="10"/>
<hc:TextBox hc:TitleElement.Title="年龄:" hc:TitleElement.TitleWidth="60" Text="{Binding Age}" Width="200" hc:TitleElement.TitlePlacement="Left" Margin="10"/>
<hc:TextBox hc:TitleElement.Title="身高:" hc:TitleElement.TitleWidth="60" Text="{Binding Height}" Width="200" hc:TitleElement.TitlePlacement="Left" Margin="10"/>
<hc:TextBox hc:TitleElement.Title="体重:" hc:TitleElement.TitleWidth="60" Text="{Binding Weight}" Width="200" hc:TitleElement.TitlePlacement="Left" Margin="10"/>
</StackPanel>
</Viewbox>
</UserControl>
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
PersonEXView的代码如下:
<UserControl x:Class="UserControlScaleDemo.View.PersonEXView"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:local="clr-namespace:UserControlScaleDemo.View"
mc:Ignorable="d"
d:DesignHeight="450" d:DesignWidth="800"
>
<Grid>
<Border BorderBrush="{StaticResource PrimaryBrush}" BorderThickness="2" />
<ContentControl>
<ContentControl.Content>
<local:PersonView/>
</ContentControl.Content>
</ContentControl>
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Left" VerticalAlignment="Top" Margin="-3,-3" Cursor="SizeNWSE" x:Name="left_top" MouseLeftButtonDown="left_top_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Center" VerticalAlignment="Top" Margin="0,-3" Cursor="SizeNS" x:Name="center_top" MouseLeftButtonDown="center_top_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Right" VerticalAlignment="Top" Margin="-3,-3" Cursor="SizeNESW" x:Name="right_top" MouseLeftButtonDown="right_top_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Right" VerticalAlignment="Center" Margin="-3,0" Cursor="SizeWE" x:Name="right" MouseLeftButtonDown="right_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Right" VerticalAlignment="Bottom" Margin="-3,-3" Cursor="SizeNWSE" x:Name="right_bottom" MouseLeftButtonDown="right_bottom_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Center" VerticalAlignment="Bottom" Margin="0,-3" Cursor="SizeNS" x:Name="bottom" MouseLeftButtonDown="bottom_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Left" VerticalAlignment="Bottom" Margin="-3,-3" Cursor="SizeNESW" x:Name="left_bottom" MouseLeftButtonDown="left_bottom_MouseLeftButtonDown" />
<Ellipse Width="7" Height="7" Fill="{StaticResource PrimaryBrush}" HorizontalAlignment="Left" VerticalAlignment="Center" Margin="-3,-3" Cursor="SizeWE" x:Name="left" MouseLeftButtonDown="left_MouseLeftButtonDown" />
</Grid>
</UserControl>
/// <summary>
/// PersonEXView.xaml 的交互逻辑
/// </summary>
public partial class PersonEXView : UserControl
{
public PersonEXView()
{
InitializeComponent();
this.DataContext = this;
}
public LayerState layerState = LayerState.None;
private void left_top_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void center_top_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void right_top_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void right_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void right_bottom_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
layerState = LayerState.RightBottom;
}
private void bottom_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void left_bottom_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
private void left_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
75
76
77
78
MainWindow的代码如下:
<Window x:Class="UserControlScaleDemo.MainWindow"
xmlns="http://schemas.microsoft.com/winfx/2006/xaml/presentation"
xmlns:x="http://schemas.microsoft.com/winfx/2006/xaml"
xmlns:d="http://schemas.microsoft.com/expression/blend/2008"
xmlns:mc="http://schemas.openxmlformats.org/markup-compatibility/2006"
xmlns:local="clr-namespace:UserControlScaleDemo"
mc:Ignorable="d"
xmlns:view="clr-namespace:UserControlScaleDemo.View"
Title="MainWindow" Height="450" Width="800"
MouseMove="Window_MouseMove"
MouseLeftButtonDown="Window_MouseLeftButtonDown"
Background="red">
<Grid>
<view:PersonEXView Height="200" Width="200"/>
</Grid>
</Window>
/// <summary>
/// Interaction logic for MainWindow.xaml
/// </summary>
public partial class MainWindow : Window
{
public MainWindow()
{
InitializeComponent();
}
private void Window_MouseMove(object sender, MouseEventArgs e)
{
if(e.LeftButton == MouseButtonState.Pressed)
{
Point p = e.GetPosition(this);
double x = p.X - pressedPoint.X;
double y = p.Y - pressedPoint.Y;
var view = GetChildData<PersonEXView>(this, p);
if (view != null)
{
switch (view.layerState)
{
case Model.LayerState.RightBottom:
view.Height += y;
view.Width += x;
break;
}
}
pressedPoint = p;
}
}
public static T GetChildData<T>(UIElement el, Point p) where T : DependencyObject
{
IInputElement obj = el.InputHitTest(p);
DependencyObject target = obj as DependencyObject;
while (target != null)
{
if (target is T)
{
T t = (T)target;
return t;
}
target = VisualTreeHelper.GetParent(target);
}
return default(T);
}
private Point pressedPoint = new Point();
private void Window_MouseLeftButtonDown(object sender, MouseButtonEventArgs e)
{
pressedPoint = e.GetPosition(this);
}
}
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40
41
42
43
44
45
46
47
48
49
50
51
52
53
54
55
56
57
58
59
60
61
62
63
64
65
66
67
68
69
70
71
72
73
74
上面的代码中只实现了右下角的缩放,其他的可以自行完善。